接口开发
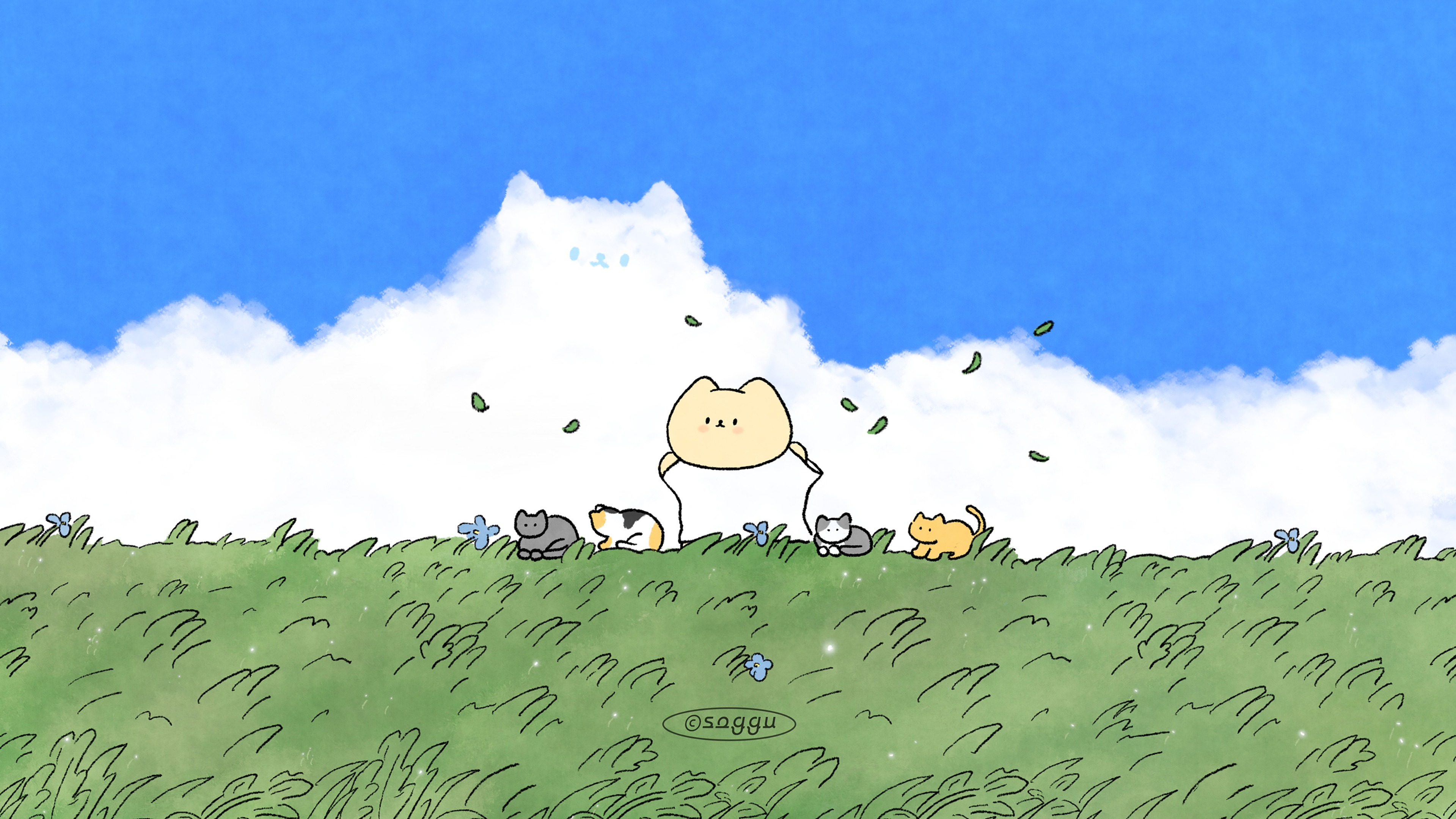
接口开发
黄文胜用户分类统计接口(10.23)
接口名称:用户分类统计接口
开发内容:这是我在实习中的第一个开发接口,主要实现用户的分类统计功能。接口通过执行 SQL 查询从数据库中获取用户信息(如用户类型和用户名),并根据用户类型进行分组统计。
具体实现步骤:
定义SQL查询
编写SQL语句:通过SQL语句查询
sm_user
表中查询用户类型和相应的用户数量。1
SELECT user_type, COUNT(*) AS user_count FROM sm_user GROUP BY user_type
执行查询
使用
BaseDAO
的executeQuery
方法执行SQL查询,并将结果转换成List<UserTypeCountVo>
对象列表,每个对象列表表示一种用户类型和相应数量,输出示例:1
2
3
4
5[
new UserTypeCountVo("admin", 20),
new UserTypeCountVo("user", 10),
new UserTypeCountVo("guest", 1)
]数据转换
将查询结果的
List<UserTypeCountVo>
列表转换为Map<String, Integer>
,其中的key为用户类型,value是对应用户的数量,便于前端解析。输出示例:1
2
3
4
5
6
7{
"data": {
"admin": 3,
"user": 5,
"guest": 2
}
}结果返回
将处理后的数据以
Map
的形式返回到data
字段中,最终作为JSON格式返回给调用者。代码实现
封装查询字段:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30// 查询用户
public static class UserTypeCountVo extends SuperVO {
// 无参构造方法
public UserTypeCountVo() {
super();
}
// 用户类型字段
private String user_type;
// 用户数量字段
private Integer user_count;
// Getter 和 Setter 方法
public String getUser_type() {
return user_type;
}
public void setUser_type(String user_type) {
this.user_type = user_type;
}
public Integer getUser_count() {
return user_count;
}
public void setUser_count(Integer user_count) {
this.user_count = user_count;
}
}执行查询,接口实现:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26public class CountUsersByTypeAction extends BaseAction {
public Object doAction(IRequest request, RequstParamWapper paramWapper) throws Throwable {
// 创建数据库操作对象
BaseDAO baseDAO = new BaseDAO();
String sql = "SELECT user_type, COUNT(*) AS user_count FROM sm_user GROUP BY user_type";
// 执行SQL查询操作,并将结果转换为List<UserTypeCountVo>
List<UserTypeCountVo> userTypeCountVos = (List<UserTypeCountVo>) baseDAO.executeQuery(sql, new BeanListProcessor(UserTypeCountVo.class));
// 构建返回的结果数据,将List<UserTypeCountVo>转换为Map<String, Integer>
Map<String, Integer> resultMap = new HashMap<>();
for (UserTypeCountVo userTypeCountVo : userTypeCountVos) {
resultMap.put(userTypeCountVo.getUser_type(), userTypeCountVo.getUser_count());
}
// 构建返回的数据
HashMap<String, Object> map = new HashMap<>();
map.put("data", resultMap);
// 返回查询结果
return map;
}
}
用户类型名字列举接口(10.25)
1 | public static class UserNameListVo extends SuperVO { |
评论
匿名评论隐私政策
TwikooWaline
✅ 你无需删除空行,直接评论以获取最佳展示效果